Introduction
Complex online projects must be able to support the great majority of users and developers. It must also handle a large number of distinct UI components. Many developers use React to create scalable web applications in these circumstances.
React is the most popular library in the JavaScript ecosystem. We are all aware of React's advantages, but do we really understand what makes it a superior option for front-end development?
Adaptability, component-based design, virtual DOM, and other optimization techniques are extremely advantageous when developing large-scale, high-traffic apps.
While the technology has proven to be advantageous for app development, various React.js app scalability concerns might still exist. So, in this blog, we will be looking at the common scalability challenges in ReactJS and its solutions.
ReactJS Scalability - Let's dive in & find out!
Here are a few common challenges and their solutions!
Problem #1: Performance Problems while application scaling!
When your ReactJS application scales in size and complexity, performance issues might arise, which impacts the end-to-end responsiveness and user experience of your application.
It also makes the rendering and re-rendering slower when the number of elements and the complexity increases - leading to a variety of performance bottlenecks in the React application while affecting the overall user experience.
Additionally, large component trees can slow down the application as rendering the entire tree becomes resource-intensive, particularly with long lists or deeply nested components.
Solution #1: Implement Memorization, Visualization & Code-splitting.
To solve these performance problems in the react js applications, you can opt for different types of solutions. All these performance optimization techniques not only enhance the scalability of your ReactJS apps but also ensure a seamless user experience when it comes to scaling your Reactjs application.
Find out some of the best practices to solve performance issues in Reactjs while scaling -
- You can go for memoization techniques such as React. Memo or React.PureComponent to prevent unnecessary re-renders.
- In this case, some of the virtualization techniques, such as React Virtualized or React Window, are also helpful as it allows rendering only the visible portion of long lists or large data sets, reducing the number of DOM elements and improving performance.
- The third & and most beneficial way of improving performance at the times of times is through code-splitting. You can do the code-splitting through Webpack or React. Lazy, which helps load code on-demand, reducing the initial bundle size and enhancing application startup time.
Problem #2: Compatibility problems while scaling the Reactjs app!
When you are looking forward to scaling your Reactjs application, you might deal with compatibility due to the usage of different libraries, dependencies, or React versions.
All these compatibility issues can result in conflicts, unexpected behavior, errors, or even application crashes. As the application grows and more developers contribute to the codebase, compatibility management also becomes increasingly challenging, especially while trying to integrate any third-party libraries or migrate to newer versions of React.
Solution #2: Comprehensive documentation, reviewing, and testing!
To address compatibility issues while scaling a ReactJS application, there are various best practices you can follow.
- Firstly, it is crucial to keep updated documentation of all the dependencies, along with their versions and any specific compatibility needs. This documentation will help Reactjs programmers ensure that they have selected libraries and versions that are compatible with each other and even with an ideal React version.
- On top of it, regularly reviewing and updating the dependencies will also help identify and resolve any compatibility problems. You should also not skip thorough testing, such as unit tests and integration tests, to analyze the compatibility of varied elements and libraries.
- We recommend opting for automated testing frameworks such as Jest and Enzyme can, which helps you effectively identify and fix compatibility errors.
- Finally, stay active in the ReactJS community and abide by the official release notes and migration guides to get analyzed the changes or deprecated, which helps in smooth transitions and better compatibility management.
Problem #3: Security challenges while Reactjs app scaling!
When scaling a ReactJS application, there are several security challenges that can arise due to the enhanced complexity and vulnerabilities in an application. As your Reactjs application scales further, it becomes even more prone to security threats, making it even more crucial to address and solve security problems.
Some of the most common security issues include cross-site scripting attacks, cross-site request forgery, insecure data handling, and unauthorized access to confidential information.
These vulnerabilities can lead to data breaches, unauthorized access, and compromised user accounts, leading to significant for users and applications as a whole.
Solution #3: Opting for a Multi-layered security approach
Opt for a multi-layered approach to address security problems when you are looking to scale your Reactjs application.
- Firstly, you need to implement secure coding practices in order to solve common security problems like XSS and CSRF.
- Use security libraries and frameworks specifically designed for ReactJS, such as Helmet, to add additional security headers and protections.
- We recommend properly handling and sanitizing user input, validating API requests, and enforcing secure communication protocols (HTTPS) to protect data in transit.
- Implement access controls and authentication mechanisms to ensure that only authorized users can access sensitive resources.
- Regularly perform security audits and penetration testing to identify and rectify potential vulnerabilities.
- Finally, stay updated with the latest security best practices and follow security guidelines given by ReactJS and related security communities to stay informed about the latest security threats and solutions.
Problem #4: Challenge of network latency while application scaling
Network latency is another sign that Reactjs applications might face while scaling. As your application scales and needs more information to be fetched from the server, network latency can occur, resulting in slower response times and a less responsive user experience.
The delay in communication between the client-side application and the server can lead to longer page loading times, delayed data updates, and reduced interactivity - which in turn leads to poor customer experience throughout.
The problem of Network latency usually occurs when you are dealing with huge data transfers or when users from different and distant geographic locations try to access your app.
Solution #4: Various effective ways to solve this Network latency problem in your Reactjs application.
If you are facing the problem of network latency while scaling your Reactjs application and are looking for effective solutions to prevent these problems, then check out the following solutions you can try to ensure a smooth user experience throughout your Reactjs application.
- Adopt the methods of data pagination and infinite scrolling to fetch and display data in smaller chunks, which helps minimize the amount of data transferred in each request.
- You can even compress and minify network payloads to optimize the data transfer size, reducing the time required for data transmission.
- Another useful approach to solve this challenge is by using client-side caching to store frequently accessed data locally. By caching data, you can fulfill the subsequent requests without having to access the server, while reducing the impact of latency.
- For pre-rendering the initial HTML on the server, implementing server-side rendering (SSR) can help as it reduces the time needed for the client to fetch and render the initial page.
- Opt for Content Delivery Network (CDN) to improve your network performance by distributing static assets closer to end-users, reducing the physical distance and network hops required to fetch resources.
Problem #5: Challenge of Memory leaks while Reactjs app scaling
Memory leaks are yet another challenging problem that businesses often encounter while dealing with Reactjs applications. A memory leak can happen when objects or resources are not properly released from memory, leading to a gradual accumulation of memory usage.
In ReactJS, memory leaks usually occur when you have not unmounted the elements properly or when event listeners or subscriptions are not properly cleaned up. As the application scales and more components are created and destroyed, memory leaks can become even more common while impacting the overall Reactjs app's performance and durability.
Memory leaks can even lead to enhanced memory consumption and slow application responsiveness while sometimes also resulting in application crashes. (This typically happens throughout long-running sessions or when dealing with large amounts of data.)
Solution #5: Effective management of component life cycle & resource cleaning-up!
To solve this problem while scaling your Reactjs application, make sure to stick to best practices for managing component lifecycles and resource cleanup.
- Utilize best-in-class lifecycle methods such as component will unmount or the use effect cleanup function to release any tied-up resources before you unmount it.
- Identifying all the relevant resources to prevent memory leaks is recommended.
- Make sure all the components are correctly unmounted when you no longer want to use them. It includes cleaning up any event listeners, subscriptions, or timers that were created within the component.
- Another helpful solution is to utilize tools and libraries that help in detecting and debugging memory leaks. For instance, you can use react developer tools which offer end-to-end insights into component hierarchies and memory usage, which further helps in identifying potential memory leaks.
- On top of it, regular monitoring and testing can help proactively identify and resolve memory leaks, ensuring the application's stability and optimal memory usage.
Problem #6: Code Management & Maintenance
Code management and maintenance can also become challenging as your ReactJS application scales. When your codebase grows larger and more complicated, it becomes more difficult to manage and maintain the code effectively.
You need to deal with diverse challenges in understanding and navigating the code while ensuring uniform code quality and standards throughout the application.
As an increasing number of developers contribute to the codebase, the risk of code duplication, inconsistencies, and changes also further doubles.
But, without proper code organization and documentation, maintaining and updating the application becomes a time-consuming and error-prone process leading to inconsistencies in an application.
Solution #6: Adopt the best code management & maintenance practices!
To address the code management and maintenance challenges in your ReactJS application while scaling, you need to abide by some of the best practices.
- Firstly, you must establish a clear and consistent code organization and structure.
- Divide the codebase into logical modules or features, and follow a modular architecture. This approach promotes code reuse and maintainability while making locating and updating specific functionality easier.
- We suggest going for intuitive naming conventions and directory structures that align with your personalized project's needs and scale.
- Regular code reviews and peer programming sessions help identify and address potential issues early on.
- We recommend documenting the purpose, functionality, and usage of key components, functions, and APIs to facilitate collaboration and knowledge sharing among the development team.
- Maintain a clean and well-structured Git workflow, utilizing branches, pull requests, and code reviews to ensure a controlled and organized development process.
- You can also benefit from the Continuous integration and continuous deployment (CI/CD) practices to streamline your code management.
By adopting these code management practices, utilizing the right appropriate tools, and fostering a culture of clean code and collaboration, you can significantly improve the maintainability of your ReactJS application.
ReactJS Scalability - Wrapping up!
Here's a complete wrap on handling the scalability challenges of Reactjs applications with effective solutions for the same. We have mentioned the problem and its solution in detail to help you cope-up with the challenges accordingly.
Although, with the right approach and best practices - Reactjs is the best choice when it comes to development and can manage scaling problems as well.
If you are still in doubt and looking for expert guidance, then reach out to Giriraj Digital - a top-notch ReactJS Development Company that helps here. We have a team of skilled Reactjs developers, who understand your needs, and problems with scaling and offer effective solutions accordingly.
Book a free consultation call with our expert right away!
Most Popular blogs
Reactjs V/s Vuejs - Check out the ultimate comparison and choose the right one!
3 Mins read
10 Things you should know when Hiring a ReactJS Development Company!
6 Mins read
NextJs: Advanced Features and Benefits for Elevated Development
7 Mins Read
Best ReactJS Libraries for Building a React Application
4 Mins read
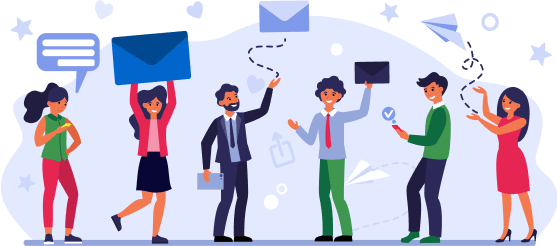
Join 1500+ people for our free Newsletter
Sign up now to receive exclusive updates, industry insights, and special offers directly to your inbox.